사진 가로와 세로 사이즈를 취득하는 방법을 알아보겠습니다.
파이썬에는 OpenCV 또는 Pillow(PIL) 등등 사진 파일에 사용할 수 있는 라이브러리가 있습니다.
OpenCV와 Pillow(PIL)를 사용해 사진 사이즈를 취득하는 예제를 보겠습니다.
OpenCV
사이즈를 취득할 때 OpenCV를 사용하면 NumPy 배열 ndarray로 취득합니다.
ndarray 형태로 취득해 shape를 사용해 사진의 가로와 세로 사이즈를 취득할 수 있습니다.
먼저 샘플 사진은 아래 이미지를 사용하겠습니다.
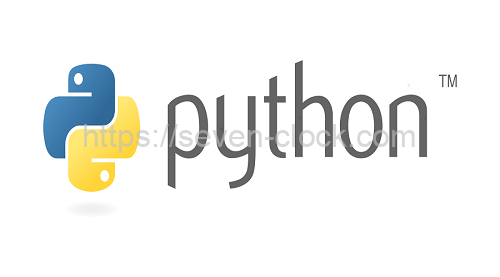
사진에서 취득하는 내용은 세로(높이) x 가로(넓이) x 색(3) 정보를 취득합니다.
import cv2
img = cv2.imread('C:/Users/Administrator/Desktop/python/origin/python.png')
print(type(img))
print(img.shape)
print(type(img.shape))
결과
<class 'numpy.ndarray'>
(277, 500, 3)
<class 'tuple'>
타입은 튜플 형태로 세로(높이) x 가로(넓이) x 색(3)를 취득해왔습니다.
값을 하나씩 변수에 담고 싶은 경우는 변수 3개를 준비해 취득할 수 있습니다.
import cv2
img = cv2.imread('C:/Users/Administrator/Desktop/python/origin/python.png')
h, w, c = img.shape
print('width: ', w)
print('height: ', h)
print('channel:', c)
결과
width: 500
height: 277
channel: 3
또는 인덱스를 설정해서도 값을 하나씩 취득할 수 있습니다.
import cv2
img = cv2.imread('C:/Users/Administrator/Desktop/python/origin/python.png')
print('width: ', img.shape[1])
print('height:', img.shape[0])
결과
width: 500
height: 277
Pillow(PIL)
사진 정보를 Pillow(PIL)를 사용해 취득하는 예제를 살펴보겠습니다.
from PIL import Image
im = Image.open('C:/Users/Administrator/Desktop/python/origin/python.png')
print(im.size)
print(type(im.size))
# (500, 277)
# <class 'tuple'>
w, h = im.size
print('width: ', w)
print('height:', h)
# width: 500
# height: 277
print('width: ', im.width)
print('height:', im.height)
# width: 500
# height: 277
Pillow(PIL)로 사진 정보를 취득하면 size 또는 width와 height로 사이즈를 취득할 수 있습니다.
size로 정보를 취득한 경우에는 튜플로 표시되기 때문에 가로와 세로 값을 따로 취득하기 위해서는 변수에 다시 저장해야 합니다.
width와 height는 가로 사이즈와 세로 사이즈를 바로 출력할 수 있습니다.
댓글